AI coding agents and AI-powered IDEs are on a path of exponential growth, with tools like Cursor, Continue.dev, and others making software development more accessible than ever. While powerful, these tools still need guidance—which is why Cursor offers cursorrules (soon to be replaced by “project rules”) to give developers more control of how the LLM interacts and generates code.
Recently, over 130 cursor rules were added in PromptHub, from this GitHub repo. I read through all of them and in this article, we’ll examine the trends, highlight interesting patterns, and offer pointers for developers using any kind of coding IDE or agent. We’ll cover:
- The most common rule categories (e.g., formatting, error handling, automation)
- Notable trends in how rules are enforced
- Unique rules that stand out, from AI etiquette to niche tech stacks
I've always found that looking at how others structure their prompts is a great way to learn!
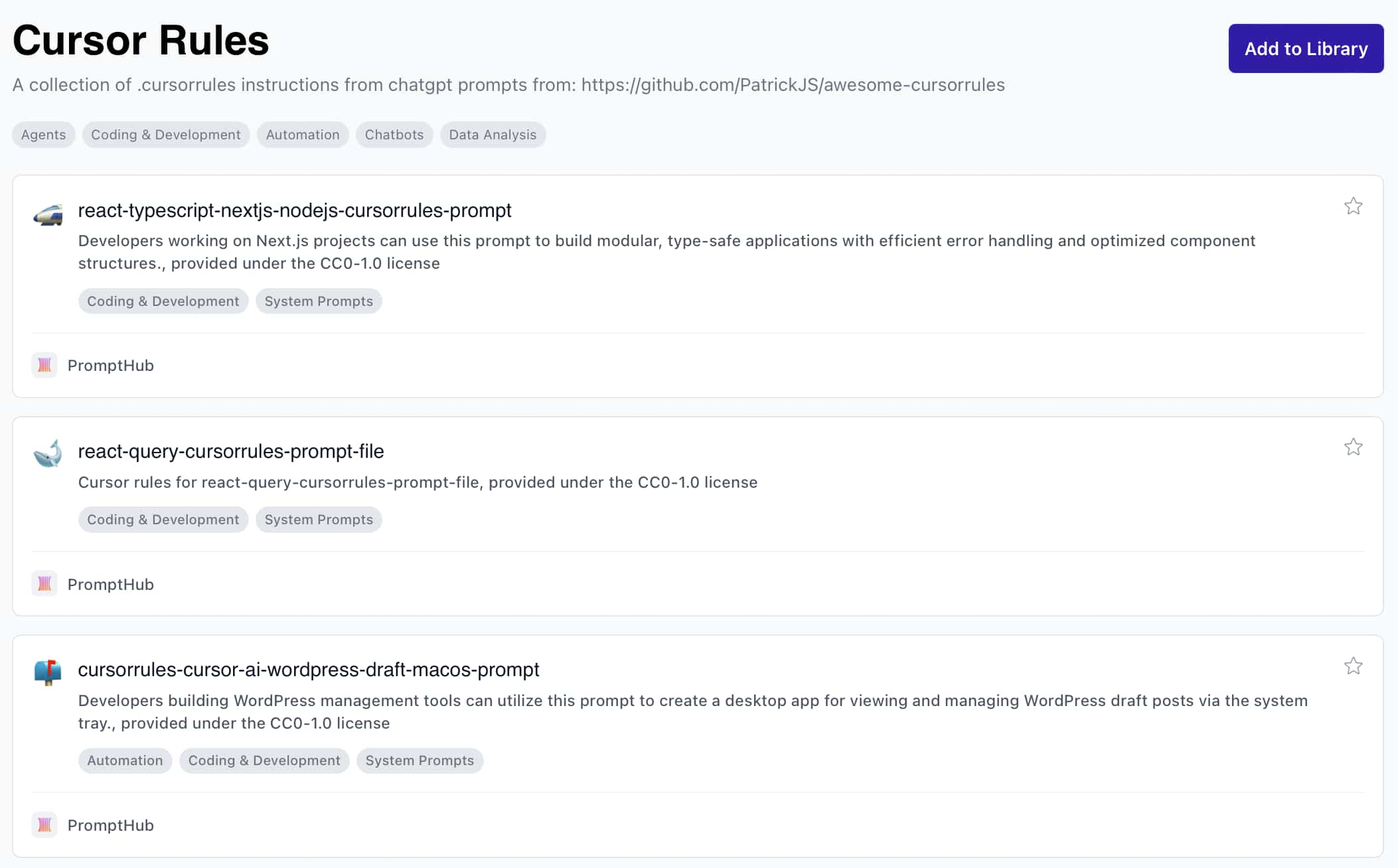
What are Cursor Rules?
Cursor rules are developer-defined coding guidelines used within Cursor.
These rules serve multiple purposes:
- Enforcing coding standards across a project.
- Improving AI-generated code quality by guiding style, formatting, and structure.
- Encouraging best practices for security, error handling, and performance.
- Reducing unnecessary code review churn by having the AI follow established team conventions.
What's cool about Cursor Rules is that they reflect real-world coding priorities, preferences, and frustrations with current models.
Common themes in Cursor Rules
Let's dive into some examples!
1. Functional programming and code structure
There is a strong preference for functional and declarative programming over traditional object-oriented patterns. Rules encourage modular, reusable code with minimal duplication.
For example, a TypeScript/Node/Next.js cursor rule states:
"Use functional and declarative programming patterns; avoid classes. Prefer iteration and modularization over code duplication. Use descriptive variable names with auxiliary verbs (e.g., isLoading, hasError)."
(Check out the full prompt in PromptHub here)
Even in frameworks where classes are necessary (like Angular), rules still promote lightweight, modular designs.
2. Consistent naming and formatting
Almost every rule set stresses consistent naming conventions and style guidelines. This includes directory and file naming, function definitions, and code organization.
For instance, front-end rules specify:
"Use lowercase with dashes for directories (e.g., components/auth-wizard). Favor named exports for components."
(Check out the full prompt in PromptHub here)
Similarly, guidelines for function definitions emphasize:
"Use the function keyword for defining pure functions. Avoid unnecessary curly braces in simple conditionals for cleaner code."
In short, consistency matters—both for readability and maintainability.
3. Type safety (TypeScript everywhere)
A major trend is strong type safety, with many rules enforcing TypeScript or equivalent type-checking tools.
"Use TypeScript for all code; prefer interfaces over types. Avoid enums; use const objects instead. Use functional components with TypeScript interfaces for props. Enable strict mode in TypeScript for better type safety."
(typescript-react-nextui-supabase-cursorrules-prompt)
Even in Python, there are recommendations to use type hints or validation tools like Pydantic, and in PHP/Laravel, to enable strict types.
4. Prioritizing error handling and validation
Handling errors early and gracefully is a major priority across the dataset. Many rules instruct developers to use guard clauses to avoid deeply nested conditionals.
"Handle errors and edge cases at the beginning of functions. Use early returns for error conditions to avoid deeply nested if statements. Place the happy path last for readability."
(cursorrules-file-cursor-ai-python-fastapi-api)
For APIs, rules recommend using custom error types and structured error responses instead of generic exceptions.
5. Testing and automation as standard practice
Testing is not optional—rules emphasize that developers should write meaningful, automated tests as part of their workflow.
One rule explicitly states:
"Ensure all tests pass before merging. Conduct thorough code reviews via pull requests; include clear PR descriptions with context. Implement comprehensive automated testing (unit, integration, e2e)."
(angular-novo-elements-cursorrules-prompt-file)
Linters and formatters are also expected to be part of the process:
"- Utilize Hardhat's testing and debugging features.
- Implement a robust CI/CD pipeline for smart contract deployments.
- Use static type checking and linting tools in pre-commit hooks."
(solidity-hardhat-cursorrules-prompt-file)
6. Performance and optimization awareness
Performance optimization is a shared responsibility.
"Use code-splitting and lazy loading for non-critical resources. Optimize images using modern formats like WebP. Prioritize Core Web Vitals (LCP, CLS, FID)."
(nextjs-vercel-typescript-cursorrules-prompt-file)
For backend services and APIs, there’s a focus on efficient queries and concurrency:
"Utilize Go’s built-in concurrency features when beneficial for API performance."
(go-servemux-rest-api-cursorrules-prompt-file)
7. Security best practices
Security is a major focus, especially in API and blockchain-related rules. A common web security guideline states:
"Validate all inputs. Implement robust authentication and authorization (OAuth 2.0, JWT). Always use HTTPS for external requests."
(python-llm-ml-workflow-cursorrules-prompt-file)
For Chrome extensions:
"Enforce Content Security Policy (CSP) in manifest.json. Use the least privilege principle for requested permissions."
(chrome-extension-dev-js-typescript-cursorrules-prompt)
These rules highlight proactive security thinking, even for everyday coding tasks.
Unique and Surprising Guidelines
While many rules reinforce industry best practices, some stood out as unique or unexpected.
1. Git commit message standards
Some rules govern commit hygiene, ensuring a structured history.
"Use Conventional Commits (feat:, fix:, docs:, chore:). Keep messages under 60 characters."
(tailwind-css-nextjs-guide-cursorrules-prompt-file)
2. Making up for AI shortcomings
Some rules guide how AI assistants should behave when generating code.
"Don't apologize for errors—fix them. If code is incomplete, add TODO comments instead."
(es-module-nodejs-guidelines-cursorrules-prompt-file)
3. Niche technology guidelines
The dataset includes rules for specialized domains, like blockchain and game development.
Example rule (for Rell, a blockchain language):
"Organize Rell code into modules. Follow SQL-like syntax patterns for querying data."
(optimize-rell-blockchain-code-cursorrules-prompt-file)
Example rule (for Chrome Extensions):
"Use chrome.alarms instead of setInterval for scheduling tasks."
(chrome-extension-dev-js-typescript-cursorrules-prompt)
Conclusion
Key takeaways for developers
- Consistency matters—style, naming, and structure should be uniform.
- Fail fast, fail clearly—catch errors early and log them properly.
- Testing and CI are mandatory—expect automation to be part of development.
- Performance and security should always be considered.
- Commit messages, AI interaction, and niche technologies have their own best practices.
Give these rules a shot in your favorite coding IDE like Cursor, Continue.dev, and others!
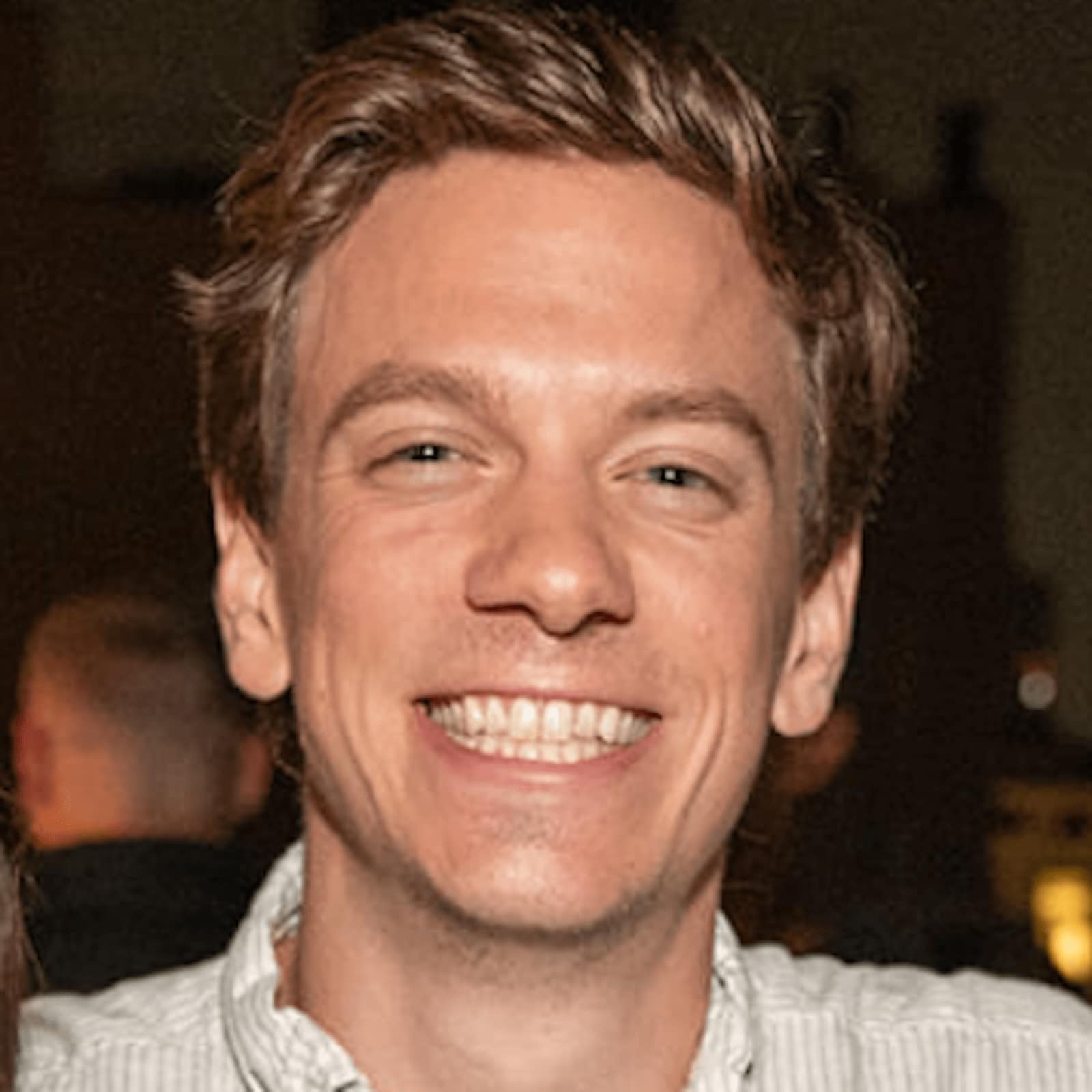